# PDF Template Manager
PDF API EXTEND PDF TEMPLATESFluent Forms PDF (opens new window) extension’s TemplateManager class allows developers to create custom PDF templates in an easy way.
You will need to extend the class TemplateManager
and push the class using the filter hook fluentform/pdf_templates
to create a new template. Please follow this documentation and create your own PDF template extension Plugin.
You can find this TemplateManager
class file in here :
plugins/fluentforms-pdf/Classes/Templates/TemplateManager.php
# Methods
# __construct()
This is the construct method of this class. You have to pass an Application object. To pass the Application object you can include it using the following namespace. Here is an example of the code so far:
use FluentForm\Framework\Foundation\Application;
use FluentFormPdf\Classes\Templates\TemplateManager;
class FluentExtraTemplateDemo extends TemplateManager
{
public function __construct(Application $app)
{
parent::__construct($app);
}
}
2
3
4
5
6
7
8
9
10
11
# getDefaultSettings()
This method will store the default settings of the PDF template. You will need to return array of the settings key & value.
public function getDefaultSettings()
{
return [
'header' => '<h2>Hello From My Demo Template</h2>',
'footer' => '<p>Footer</p>',
'body' => 'Hello There',
'demo' => ''
];
}
2
3
4
5
6
7
8
9
# getSettingsFields()
This method will render input fields for the PDF template settings page, based on the returned data. The key value used in these fields needs to match with the getDefaultSettings()
You can use the prebuilt input components to create your settings page, here is a documentation link (opens new window) where you can find the details list about these input component.
Required properties for the fields array:
key
: Settings Unique Keylabel
: Settings Input Labelcomponent
: Pass to prebuild input component.
Here is example of this method:
public function getSettingsFields()
{
return array(
[
'key' => 'header',
'label' => 'Header Content',
'tips' => 'Write your header content which will be shown every page of the PDF',
'component' => 'text'
],
[
'key' => 'body',
'label' => 'PDF Body Content',
'tips' => 'Write your Body content for actual PDF body',
'component' => 'wp-editor'
],
[
'key' => 'footer',
'label' => 'Footer Content',
'tips' => 'Write your Footer content which will be shown every page of the PDF',
'component' => 'wp-editor'
],
[
'key' => 'demo',
'label' => 'Demo Input',
'tips' => 'Input Help Text',
'component' => 'text'
],
);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
The filed tips
will show the additional help text on hover, this method is almost identical to getSettingsFields()
in the Integration Manager Controller Class.
Here is a screenshot of the above code :
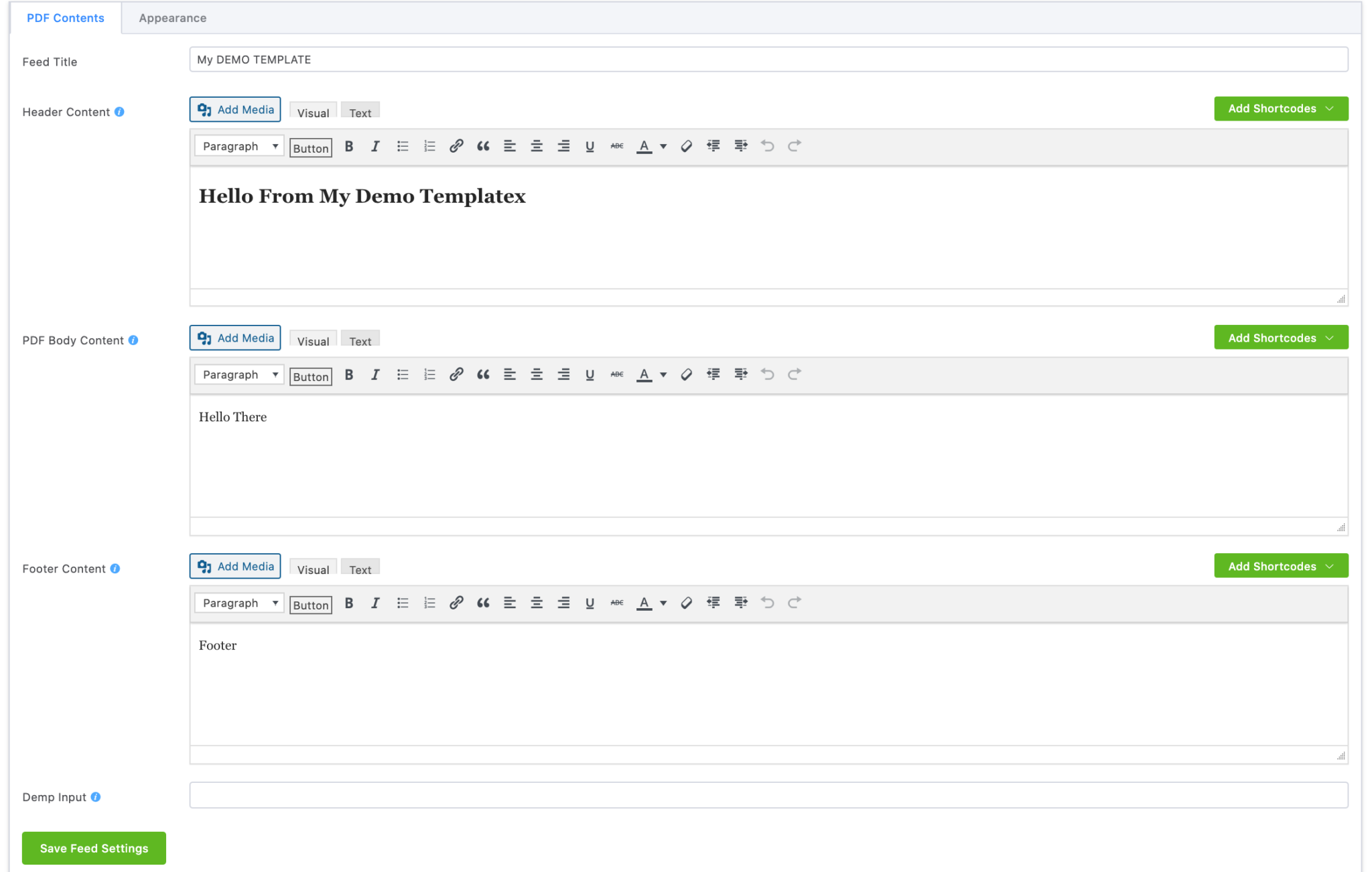
# generatePdf()
This is the most important method where the PDF will be generated. You will get four parameters here :
$submissionId
: Current submission ID$feed
: PDF feed settings$outPut = 'I'
: Do not change this$fileName = ''
: File name
The $submissionId
will contain the current submission ID. $feed
contains array data of the PDF template feed settings. This will store the input data which was generated from getSettingsFields() method. $fileName
will be the generated PDF filename.
Do not change the value of the $outPut = 'I'
Finally, you will need to call a pre-built function pdfBuilder()
and return it to create PDF file. This method needs four parameters to be passed , these are :
$fileName
: PDF File name$feed
: PDF Feed Parameter$htmlBody
: The HTML body and style of your PDF template$footer
: PDF Footer$outPut
: Output Parameter
Do not add pdf extension (.pdf) in the file name, it will be done automatically.
return $this->pdfBuilder($fileName, $feed, $htmlBody, $footer, $outPut);
The HTML can be created by fetching the user submitted form data and your custom data. Here is an example :
public function generatePdf($submissionId, $feed, $outPut = 'I', $fileName = '')
{
$submission = wpFluent()->table('fluentform_submissions') //submission data object
->where('id', $submissionId)
->first();
$htmlBody = '<h1>My PDF body</h1>'; // Html Body
$footer = $submission->created_at; // footer
$fileName = 'my-pdf-'.$submissionId; // filename
return $this->pdfBuilder($fileName, $feed, $htmlBody, $footer, $outPut);
}
2
3
4
5
6
7
8
9
10
11
12
# All Together
A demo TemplateManager class will look like this :
use FluentForm\Framework\Foundation\Application;
use FluentFormPdf\Classes\Templates\TemplateManager;
class FluentExtraTemplateDemo extends TemplateManager
{
public function __construct(Application $app)
{
parent::__construct($app);
}
public function getDefaultSettings()
{
return [
'header' => '<h2>Hello From My Demo Template</h2>',
'footer' => '<p>Footer</p>',
'body' => 'Hello There',
];
}
public function getSettingsFields()
{
return array(
[
'key' => 'header',
'label' => 'Header Content',
'tips' => 'Write your header content which will be shown every page of the PDF',
'component' => 'wp-editor'
],
[
'key' => 'body',
'label' => 'PDF Body Content',
'tips' => 'Write your Body content for actual PDF body',
'component' => 'wp-editor'
],
[
'key' => 'footer',
'label' => 'Footer Content',
'tips' => 'Write your Footer content which will be shown every page of the PDF',
'component' => 'wp-editor'
],
[
'key' => 'demo',
'label' => 'Demo Input',
'tips' => 'Input Help Text',
'component' => 'text'
],
);
}
public function generatePdf($submissionId, $feed, $outPut = 'I', $fileName = '')
{
$submission = wpFluent()->table('fluentform_submissions') //submission data
->where('id', $submissionId)
->first();
$htmlBody = '<h1>My PDF Body & other elements</h1>'; // Html Body
$footer = $submission->created_at; // footer
$fileName = 'my-pdf-'.$submissionId; // filename
return $this->pdfBuilder($fileName, $feed, $htmlBody, $footer, $outPut);
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
# Adding the Template extension as a Plugin
After completing the template class you need to push it into the PDF template list. You can use the fluentform/pdf_templates
filter hook to push the class. This can be done by creating a new plugin or inside your function.php
file.
You will get 2 parameters in the fluentform/pdf_templates
filter.
$templates
(Array) Template class & configuration listkey
: template_keyname
: Template nameclass
: Template classkey
: template_keypreview
: Template preview image location
$form
(Object) The Form Object
Here is an example of how we can create a new plugin and add the template you created. Here FluentExtraTemplateDemo.php
file is the file name that you will create.
add_action('plugins_loaded', function () {
if(!defined('FLUENTFORM_PDF_VERSION')) {
return; //checking if fluent form is activated otherwise do nothing
}
include 'FluentExtraTemplateDemo.php'; // including the template class you just created
add_filter('fluentform/pdf_templates', function ($templates, $form) {
$templates['demo_template'] = [
'name' => 'My DEMO TEMPLATE',
'class' => '\FluentExtraTemplateDemo',
'key' => 'demo_template',
'preview' => get_template_directory_uri(). 'assets/images/tabular.png'
];
return $templates;
}, 10, 2);
}, 11);
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
You can include more classes and add new templates using this method. If you have any query feel free to reach our support team (opens new window) or ask questions in our facebook community group (opens new window). Also do not forget to share your thoughts on this documentation, by adding your comment or a click on the icons below.